Supercharging Laravel with AI: Exploring Prism PHP
If you've been toying with the idea of bringing AI features into your Laravel app—whether for content generation, smart assistants, or even structured data output—Prism PHP might just be the tool you're looking for.
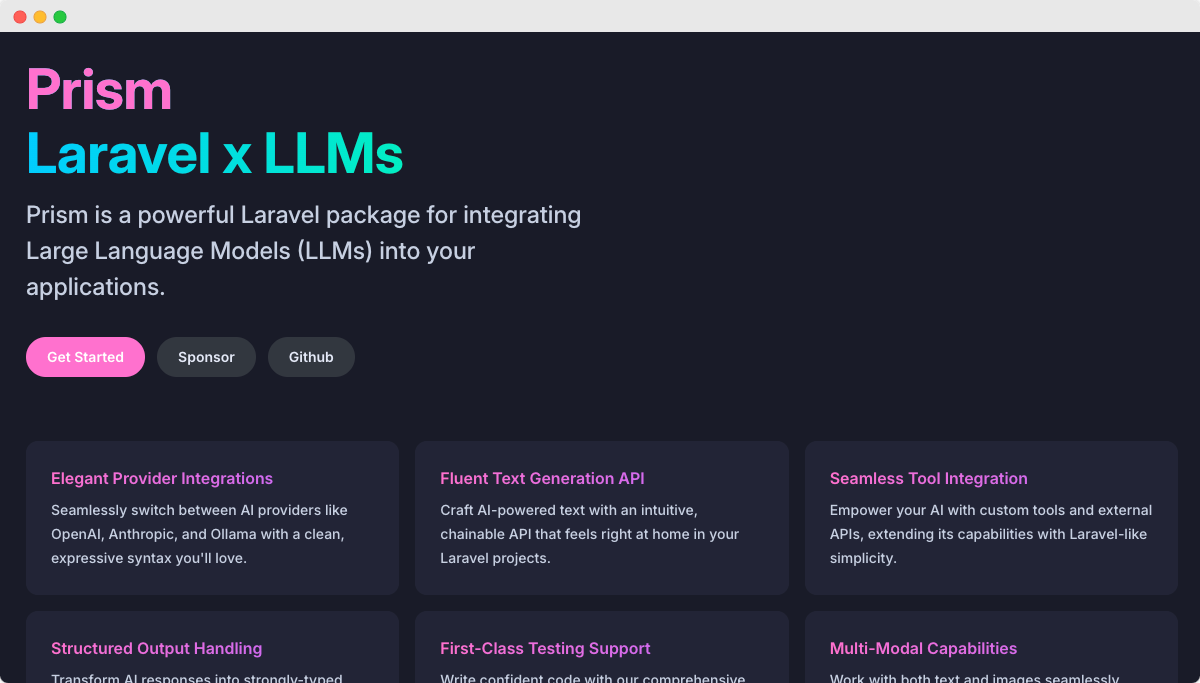
I recently took it for a spin and was genuinely impressed with how elegantly it slots into the Laravel ecosystem. If you're already comfortable with Laravel's expressive syntax and fluent interfaces, you’ll feel right at home with Prism.
What is Prism?
Prism is a Laravel package that acts as a unified wrapper for multiple Large Language Model (LLM) providers like OpenAI, Anthropic, and even local models via Ollama. Instead of locking your app into a single AI provider’s SDK or API, Prism abstracts the whole thing away—making it simple to switch providers or update models without rewriting core logic.
It's essentially AI for Laravel, done the Laravel way.
Why You Might Want to Use It
Here are a few reasons why Prism stood out to me:
- Provider-agnostic: Switch between OpenAI, Anthropic, and Ollama effortlessly.
- Fluent syntax: Works just like other Laravel services.
ThinkMail::to()->send()
but for AI prompts. - Tooling support: You can create your own tools that the AI can invoke—great for integrating custom APIs.
- Structured output: Get validated, strongly typed responses—ideal for content creation, form generation, or any structured task.
- Test-friendly: Prism offers first-class support for faking responses and asserting interactions.
Let’s look at some quick examples to give you a taste of what it’s like in practice.
Example 1: Simple Text Generation
Want to generate some AI-powered text? Here's how easy it is:
use Prism\Prism\Prism;
use Prism\Prism\Enums\Provider;
$response = Prism::text()
->using(Provider::OpenAI, 'gpt-4')
->withPrompt('Explain quantum computing to a 5-year-old.')
->asText();
echo $response->text;
It's that clean. You define your provider and model, pass in a prompt, and get your response.
Example 2: Custom Tools (Function Calling)
Prism lets you define "tools"—essentially named functions that the AI can call. Here’s an example using a fake weather service:
use Prism\Prism\Facades\Tool;
$weatherTool = Tool::as('weather')
->for('Get current weather conditions')
->withStringParameter('city', 'The city to get weather for')
->using(function (string $city): string {
return "The weather in {$city} is sunny and 72°F.";
});
Then use it in a prompt:
$response = Prism::text()
->using(Provider::Anthropic, 'claude-3-5-sonnet-latest')
->withMaxSteps(2)
->withPrompt('What is the weather like in Paris?')
->withTools([$weatherTool])
->asText();
echo $response->text;
Now you're giving the AI the ability to interact with your app’s logic or services. That’s powerful stuff.
Example 3: Structured Output with Schemas
You can also validate and structure AI output using schemas. This is super helpful for predictable content generation:
use Prism\Prism\Schemas\Schema;
$schema = Schema::object()
->property('title', Schema::string())
->property('description', Schema::string());
$response = Prism::text()
->using(Provider::OpenAI, 'gpt-4')
->withPrompt('Generate a blog post title and description about AI in healthcare.')
->withSchema($schema)
->asStructured();
echo $response->title;
echo $response->description;
This turns your AI response into a well-structured object—great for templating, APIs, or saving into the database.
Final Thoughts
Prism really lowers the barrier for Laravel devs to start experimenting with AI. The API is expressive and predictable, the provider abstraction is solid, and the tooling is already mature enough for production work.
If you're looking to add AI features to your Laravel project—whether for content, chatbots, automation, or data transformation—Prism is absolutely worth a look.
You can dive into the full docs at prismphp.com or check out the GitHub repo.
Have you used AI in a Laravel app yet? Drop a message on Pinkary or tweet at me—I'd love to see how others are integrating it.